Add Keyboard Shortcuts to Your Flutter App (No Plugins Needed)
In this guide, I break down Shortcuts, Actions, and Focus, and show how to wire them up in just a few lines of code.
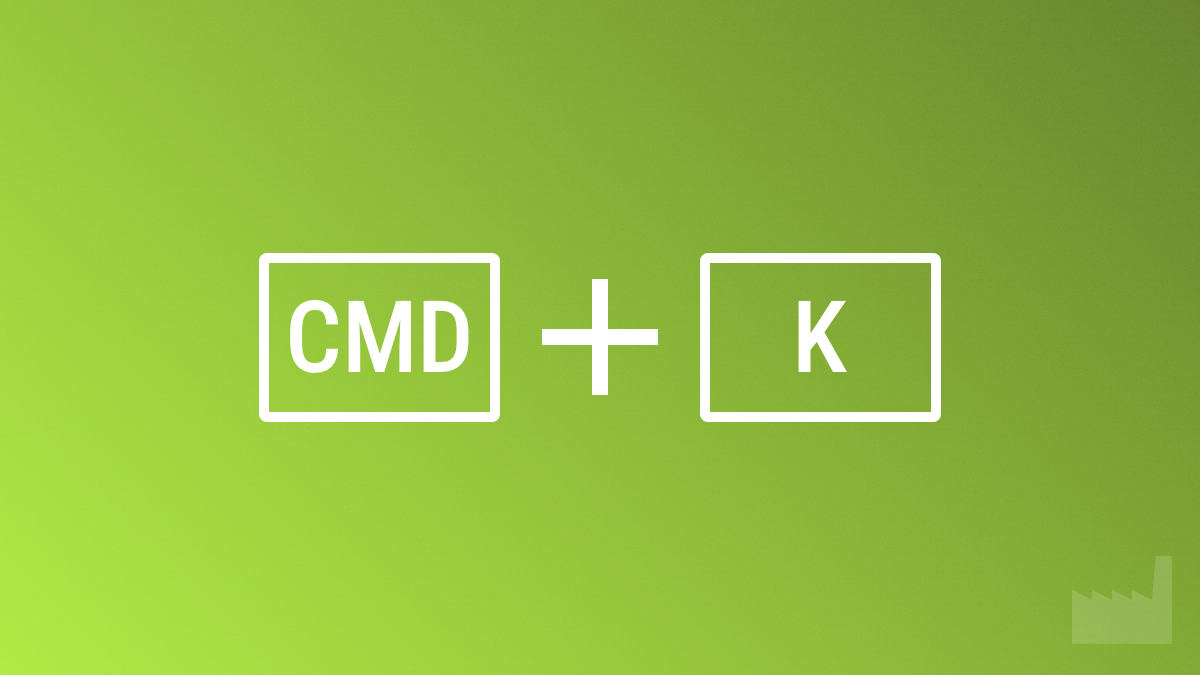
Flutter is well known for its mobile-first capabilities, but as it expands into web and desktop, user expectations are shifting. Users on these platforms expect familiar, keyboard-driven experiences: pressing Ctrl+S
to save, Ctrl+F
to search, or even arrow keys for navigation.
What many Flutter developers don’t realize is that Flutter has first-class support for keyboard shortcuts built right into the framework, no plugins required. In this article, we’ll walk through how to implement keyboard shortcuts using Shortcuts
, Actions
, and Focus
, all from the Flutter SDK.
Whether you’re building a productivity tool, admin panel, or content editor, this guide will help you add a layer of polish that makes your app feel native.
Why Keyboard Shortcuts Matter
Keyboard shortcuts are often overlooked, but they:
- Improve usability for power users
- Boost accessibility
- Enhance perceived performance (faster than clicking through menus)
- Help your app feel more like a real desktop application
Let’s explore how you can add them to your Flutter app in just a few minutes.
Step 1: Understand the Building Blocks
Flutter’s shortcut system is built around three core widgets:
Shortcuts
Maps a combination of keyboard keys to an Intent
. Think of Intent
as a label for the desired action.
Actions
Defines what should happen when a specific Intent
is triggered. You bind actions to the matching Intent
types.
Focus
Needed to capture keyboard input. Without focus, the widget tree won’t respond to keyboard events.
These widgets can be composed around any part of your widget tree, but for global shortcuts, they should wrap your main content.
Step 2: Implement Your First Shortcut
Let’s build a small example: when the user presses Ctrl+S
, show a SnackBar; Ctrl+F
opens a dialog; and Right Arrow
logs an action.
Example Code
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Shortcuts Demo',
theme: ThemeData(primarySwatch: Colors.blue),
home: const Scaffold(body: ShortcutExample()),
);
}
}
class ShortcutExample extends StatelessWidget {
const ShortcutExample({super.key});
@override
Widget build(BuildContext context) {
return Shortcuts(
shortcuts: <LogicalKeySet, Intent>{
LogicalKeySet(LogicalKeyboardKey.keyS, LogicalKeyboardKey.control): const SaveIntent(),
LogicalKeySet(LogicalKeyboardKey.keyF, LogicalKeyboardKey.control): const SearchIntent(),
LogicalKeySet(LogicalKeyboardKey.arrowRight): const NextIntent(),
},
child: Actions(
actions: <Type, Action<Intent>>{
SaveIntent: CallbackAction<SaveIntent>(onInvoke: (intent) {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(content: Text('Save triggered')),
);
return null;
}),
SearchIntent: CallbackAction<SearchIntent>(onInvoke: (intent) {
showDialog(
context: context,
builder: (context) => const AlertDialog(
content: Text('Search triggered'),
),
);
return null;
}),
NextIntent: CallbackAction<NextIntent>(onInvoke: (intent) {
debugPrint('Next item triggered');
return null;
}),
},
child: Focus(
autofocus: true,
child: const Center(
child: Text('Press Ctrl+S to Save, Ctrl+F to Search, or → to go Next.'),
),
),
),
);
}
}
class SaveIntent extends Intent {
const SaveIntent();
}
class SearchIntent extends Intent {
const SearchIntent();
}
class NextIntent extends Intent {
const NextIntent();
}
Step 3: Break It Down
Let’s explain what’s happening here:
Shortcuts
widget defines keyboard mappings: key combinations →Intent
classes.Actions
widget maps eachIntent
to a handler function viaCallbackAction
.Focus
ensures that the widget tree is listening for keyboard events.
These three work together like a controller pattern: input (keys) → intent → action.
If you don’t add Focus
or forget autofocus: true
, nothing will respond.
Step 4: Add More Shortcuts
You can easily add more mappings:
LogicalKeySet(LogicalKeyboardKey.keyK, LogicalKeyboardKey.meta): const CommandPaletteIntent(),
Use LogicalKeyboardKey.meta
for Cmd on macOS or the Windows key.
You can even handle combinations like Shift + Ctrl + R
:
LogicalKeySet(
LogicalKeyboardKey.shift,
LogicalKeyboardKey.control,
LogicalKeyboardKey.keyR
): const RefreshIntent(),
Step 5: Use This in Your Real App
Keyboard shortcuts are useful in a wide variety of use cases:
- Productivity Tools: Save, search, navigate tabs
- Note-taking Apps: Toggle preview modes, insert timestamps
- Admin Panels: Quick add, refresh tables, open modals
- Content Editors: Cmd+B for bold, Cmd+I for italics
You can combine this with FocusTraversalGroup
or FocusNode
to build full keyboard navigation experiences.
Bonus: Scoped vs Global Shortcuts
By wrapping only parts of your widget tree in Shortcuts
, you can make shortcut behavior contextual. For example, maybe Enter
submits a form only when a text field has focus. Or Escape
only closes a dialog when it's visible.
Flutter’s shortcut system is flexible enough to support both global and scoped use.
Gotchas to Watch Out For
- Shortcuts only work when the
Focus
tree is active. Always useautofocus: true
or manage focus explicitly. - Some shortcuts (like
Cmd+R
in Flutter Web) are intercepted by the browser. Avoid conflicts with browser-reserved keys. - Keyboard shortcuts don’t work well on mobile, consider using platform checks if needed.
Wrapping Up
Keyboard shortcuts are a small feature with a big impact, especially for web and desktop apps. With just a few widgets: Shortcuts
, Actions
, and Focus
, you can deliver a smooth, intuitive, keyboard-friendly experience.
The best part? No extra dependencies, and complete control.
Whether you’re building a Markdown editor, a custom dashboard, or a power-user tool, keyboard shortcuts help you build apps that feel right.
Give it a try, your future users will thank you.
Looking forward to your feedback, as always.
Get the Code
You can explore, run, and modify the example project by cloning the repository:
git clone https://github.com/dartfoundry/flutter-shortcut-example
Or visit the DartFoundry GitHub repo.